URL路由系统是什么
简而言之,路由系统就是URL路径和视图函数的一个对应关系,也可以称为转发器。
URL配置
URL路由系统格式:
urlpatterns = [ path(regex, view, kwargs=None, name=None) ]
|
urlpatterns:一个列表,每一个path()函数是一个元素,对应一个视图
参数:
URL路由分发
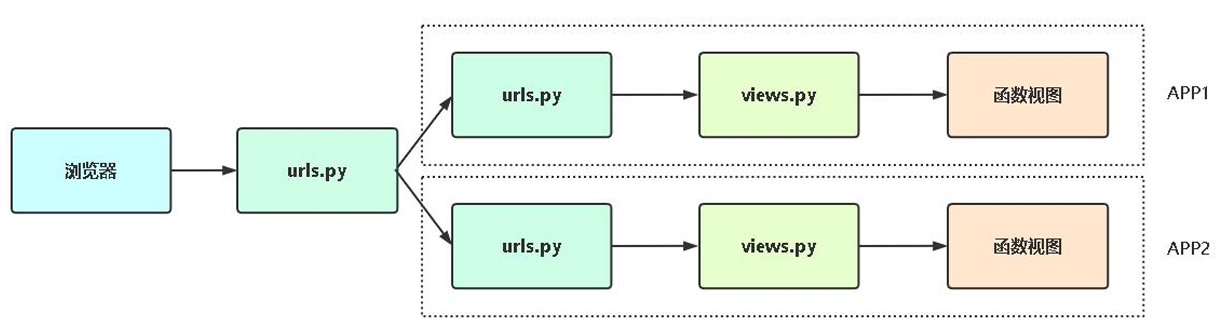
URL路由分发好处:urls配置解耦,方便管理
示例:
from myapp import views as myapp_views
urlpatterns = [ path('index', views.index), path('myapp/', include('myapp_views.urls')), ]
urlpatterns = [ path("hello", views.hello) ]
|
访问地址:http://127.0.0.1:8000/myapp/hello
URL正则表达式匹配
URL路径也可以使用正则表达式匹配,re_path()替代path()
示例:博客文章归档访问形式
from django.urls import re_path from devops import views
urlpatterns = [ re_path('articles/2020/$', views.specified_2020), re_path('^articles/([0-9]{4})/$', views.year_archive), re_path('^articles/([0-9]{4})/([0-9]{2})/$', views.month_archive), re_path('^articles/([0-9]{4})/([0-9]{2})/([0-9]+)/$', views.article_detail), ]
|
视图:
def specified_2020(request): return HttpResponse("指定2020年 文章列表") def year_archive(request, year): return HttpResponse("%s年 文章列表" % year) def month_archive(request, year, month): return HttpResponse("%s年/%s月 文章列表" % (year, month)) def article_detail(request, year, month, id): return HttpResponse("%s年/%s月 文章ID: %s" %(year, month, id))
|
命名分组语法:(?Ppattern) 其中name是名称,pattern是匹配的正则表达式
示例:博客文章归档访问形式
from django.urls import re_path from devops import views
urlpatterns = [ re_path('articles/2020/$', views.specified_2020), re_path('^articles/(?P<year>[0-9]{4})/$', views.year_archive), re_path('^articles/(?P<year>[0-9]{4})/(?P<month>[0-9]{2})/$', views.month_archive), re_path('^articles/(?P<year>[0-9]{4})/(?P<month>[0-9]{2})/(?P<id>[0-9]+)/$', views.article_detail), ]
|
URL名称
在前端代码里经常会指定URL,例如超链接,提交表单等,这时用URL反查就方便多了。
之前: <a href="/hello">您好</a> 之后: <a href="{% url 'hello' %}">您好</a>
|